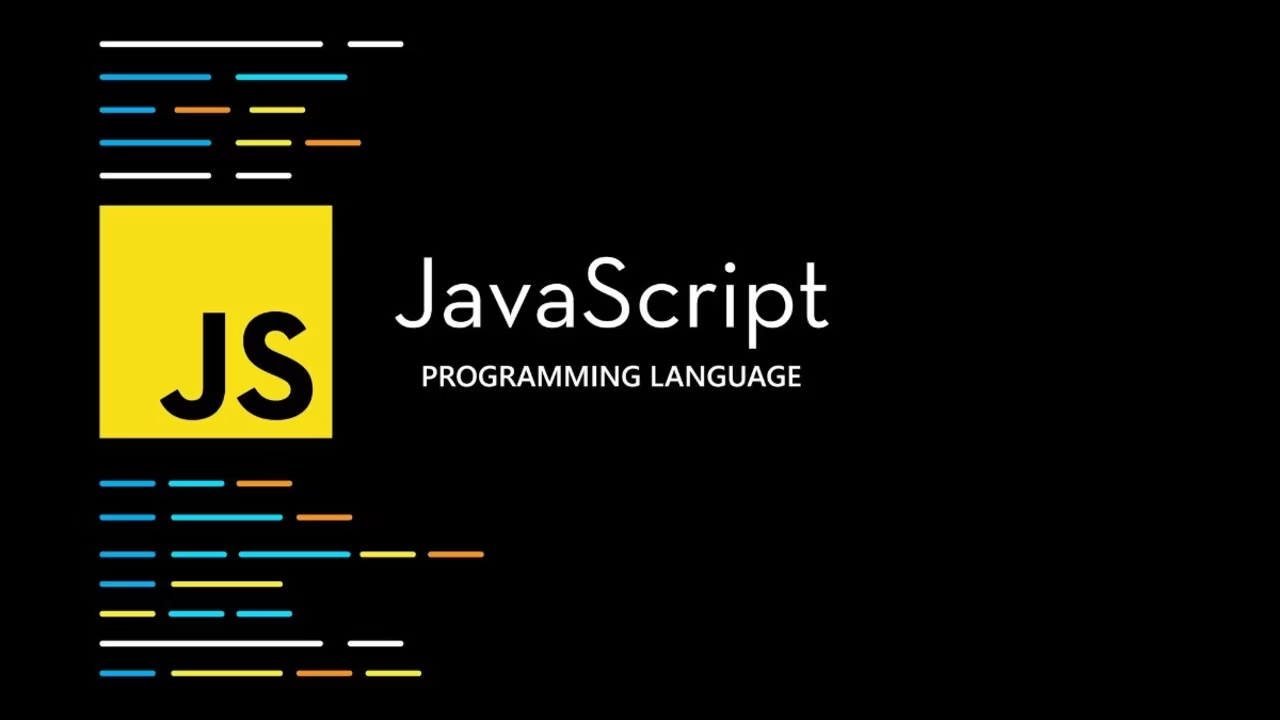
5 Essential Concepts to Master in JavaScript: MTA Skills
So, you’ve got a handle on the basics of JavaScript. You can manipulate the DOM, write functions, and maybe even wrangle a few asynchronous operations. But you’re feeling a pull, a desire to delve deeper, to unlock the true power of this versatile language. You’re ready to move beyond the fundamentals and explore the exciting world of advanced JavaScript. Great! This post, brought to you by MTA Skills, highlights five key concepts that will elevate your skills and transform you into a true JavaScript ninja. These are the skills we focus on in our advanced JavaScript training courses.
1. The Enigmatic Power of Closures:
Closures are a cornerstone of JavaScript, often misunderstood but incredibly powerful. Imagine a function nestled inside another, like a secret within a secret. The inner function, even after the outer function has finished its work, retains access to the variables of its surrounding scope. This “memory” is what makes closures so special. They enable data encapsulation (keeping variables private), create function factories (generating specialized functions), and are the foundation of many design patterns. At MTA Skills, we dedicate a significant portion of our training to understanding and applying closures.
function outerFunction() {
let message = "Hello from the outer function!";
function innerFunction() {
console.log(message);
}
return innerFunction; // Return the inner function
}
const myClosure = outerFunction(); // Now myClosure holds the inner function
myClosure(); // Output: Hello from the outer function!
2. Conquering Asynchronous Programming:
JavaScript is single-threaded, meaning it executes code line by line. But what happens when you need to fetch data from a server or handle user input? Blocking the main thread would lead to a frozen, unresponsive user experience. This is where asynchronous programming comes in. Callbacks were the original approach, but they can lead to “callback hell.” Modern JavaScript offers cleaner solutions: Promises and async/await. Promises represent the eventual result of an asynchronous operation, while async/await makes asynchronous code look and behave more like synchronous code, dramatically improving readability. MTA Skills training provides hands-on experience with Promises and async/await.
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
return data;
} catch (error) {
console.error("Error fetching data:", error);
}
}
fetchData().then(data => console.log("Data received:", data));
3. Unraveling Prototype Inheritance:
JavaScript’s inheritance model is prototype-based, a departure from the classical class-based inheritance found in languages like Java or C++. Every object in JavaScript has a prototype, which is another object. Objects inherit properties and methods from their prototypes, forming a prototype chain. Understanding this mechanism is essential for working effectively with objects and creating reusable code. Our training at MTA Skills demystifies prototype inheritance, giving you a solid foundation.
function Animal(name) {
this.name = name;
}
Animal.prototype.speak = function() {
console.log("Generic animal sound");
};
function Dog(name) {
Animal.call(this, name); // Calling Animal constructor to set the name
}
Dog.prototype = Object.create(Animal.prototype); // Inheriting from Animal
Dog.prototype.speak = function() { // Overriding the speak method
console.log("Woof!");
};
const myDog = new Dog("Buddy");
myDog.speak(); // Output: Woof!
4. Embracing Functional Programming:
Functional programming is a paradigm that emphasizes pure functions (functions without side effects), immutability (data that cannot be changed), and higher-order functions (functions that operate on other functions). JavaScript, while not a purely functional language, supports these concepts, allowing you to write more modular, testable, and maintainable code. Explore methods like map
, filter
, and reduce
to unlock the power of functional programming. MTA Skills incorporates functional programming principles into our curriculum.
const numbers = [1, 2, 3, 4, 5];
const doubled = numbers.map(x => x * 2); // Transform each element
const evenNumbers = numbers.filter(x => x % 2 === 0); // Filter for even numbers
const sum = numbers.reduce((acc, x) => acc + x, 0); // Calculate the sum
console.log(doubled); // [2, 4, 6, 8, 10]
console.log(evenNumbers); // [2, 4]
console.log(sum); // 15
5. Mastering Modules and Tooling:
As your projects grow, organization becomes crucial. Modules allow you to break down your code into reusable units, improving maintainability and collaboration. JavaScript has evolved from CommonJS to AMD, and now ES modules are the modern standard. Combine this with package managers like npm or yarn to manage dependencies, bundlers like Webpack or Parcel to optimize your code for production, and transpilers like Babel to ensure cross-browser compatibility. These tools are essential for building modern JavaScript applications. MTA Skills training covers the latest in JavaScript tooling and module management.
The Journey Continues at MTA Skills:
These five concepts are just the beginning of your journey into advanced JavaScript. There’s a vast landscape of topics to explore, from performance optimization and design patterns to metaprogramming and beyond. Embrace the challenge, keep learning, and you’ll be well on your way to becoming a JavaScript master. MTA Skills is here to guide you on that journey. Contact us today to learn more about our advanced JavaScript training programs and take your skills to the next level!