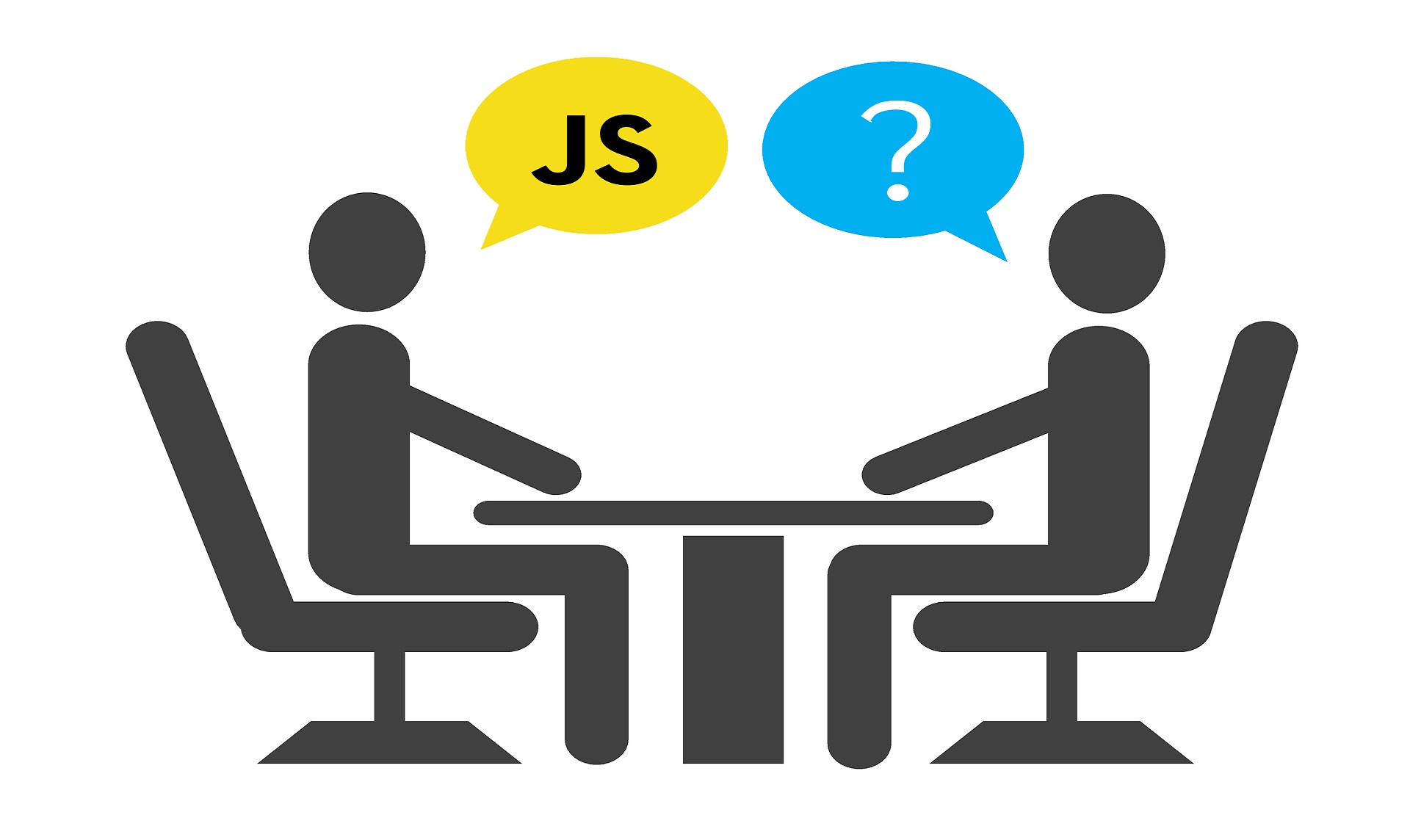
50 Most Asked JavaScript Interview Questions and Answers (2025) – MTA Skills
This blog post offers a comprehensive guide to the most frequently asked JavaScript interview questions, providing detailed answers to help you to prepare for your 2025 job interviews.
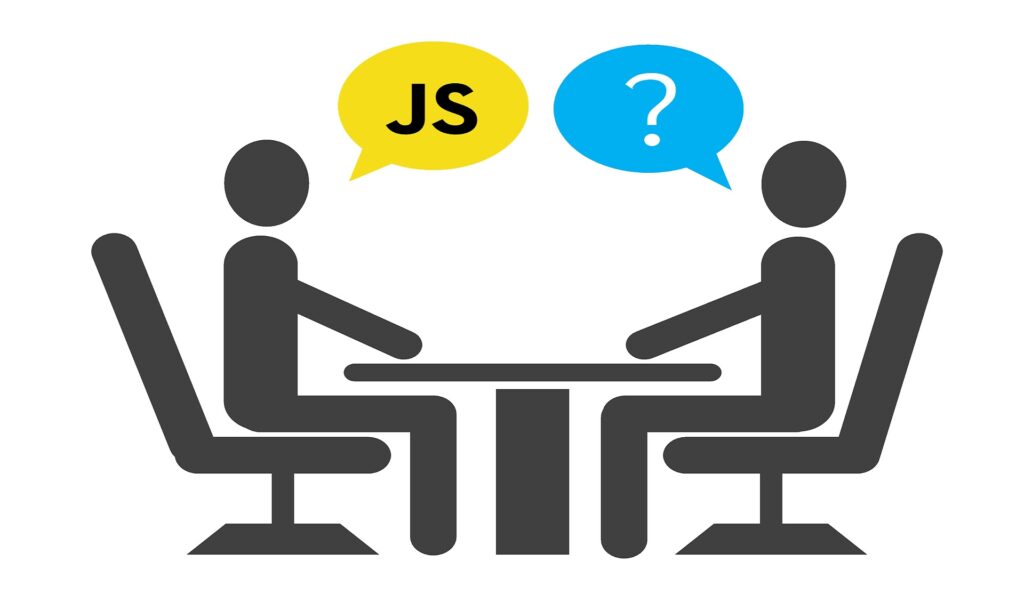
JavaScript interview can be a daunting task, with the rapid evolution of web technologies. This blog aims to provide a curated list of the most commonly asked questions in recent interviews. From fundamental concepts to advanced topics, to equip you with the knowledge and confidence to ace your career. So, lets dive in.
1. What are callback functions and how are they used?
A callback function is a function passed into another function as an argument, which is then invoked inside the outer function to complete some kind of routine or action. They are commonly used for asynchronous operations like handling events, making API calls, or reading files.
Example:
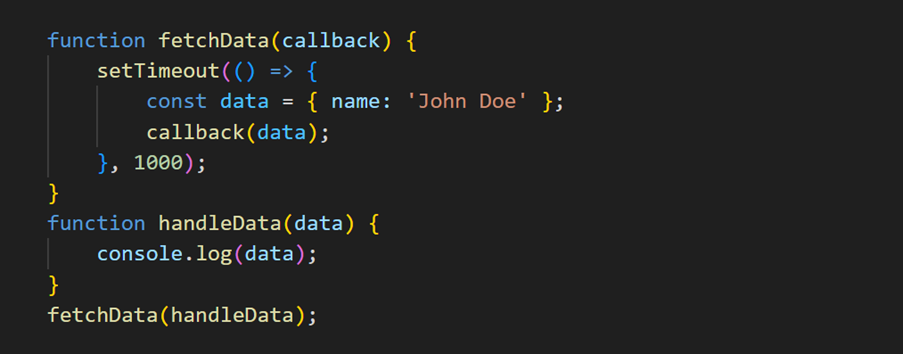
2. What’s a typical use case for anonymous functions in JavaScript?
Anonymous function in Javascript is a function that does not have any name associated with it. They are typically used as arguments to other functions or assigned to variables.

3. What are default parameters and how are they used?
Default parameters in JavaScript allow you to set default values for function parameters if no value or undefined is passed. This helps avoid undefined values and makes your code more robust. You can define default parameters by assigning a value to the parameter in the function definition.

4. Explain the difference between dot notation and bracket notation for accessing object properties.
Dot notation and bracket notation are two ways to access properties of an object in JavaScript. Dot notation is more concise and readable but can only be used with valid JavaScript identifiers. Bracket notation is more flexible and can be used with property names that are not valid identifiers, such as those containing spaces or special characters.
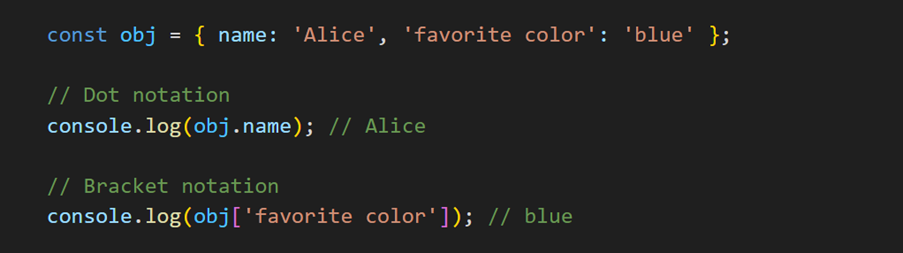
5. What are the different methods for iterating over an array?
There are several methods to iterate over an array in JavaScript. The most common ones include
- for loops
- forEach
- map
- filter
- reduce
6. How do you add, remove, and update elements in an array?
To add elements to an array, you can use methods like push, unshift, or splice. To remove elements, you can use pop, shift, or splice. To update elements, you can directly access the array index and assign a new value.
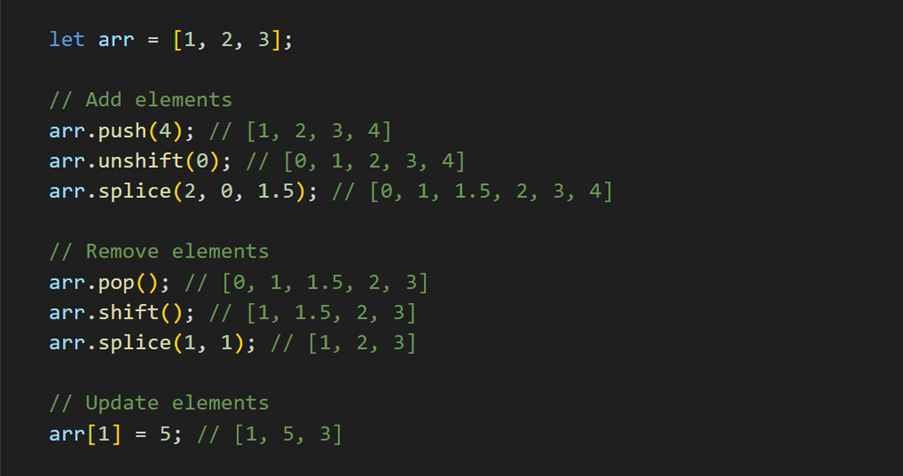
7. What are the advantages of using the spread operator with arrays and objects?
The spread operator (…) in JavaScript allows you to easily copy arrays and objects, merge them, and add new elements or properties. It simplifies syntax and improves readability. For arrays, it can be used to concatenate or clone arrays. For objects, it can be used to merge objects or add new properties.
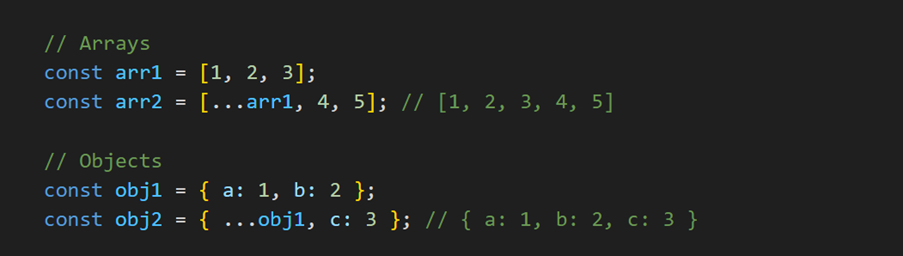
8. How do you check if an object has a specific property?
To check if an object has a specific property, you can use the in operator or the hasOwnProperty method. The in operator checks for both own and inherited properties, while hasOwnProperty checks only for own properties
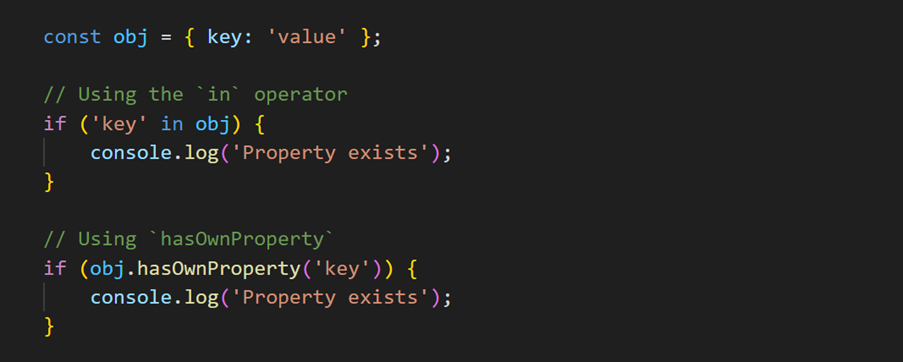
9. Explain the difference between mutable and immutable objects in JavaScript.
Mutable objects allow for modification of properties and values after creation, which is the default behaviour for most objects.
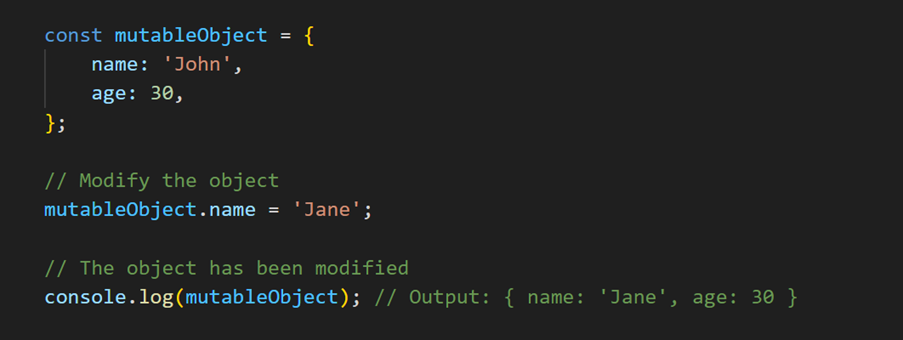
Immutable objects cannot be directly modified after creation. Its content cannot be changed without creating an entirely new value.
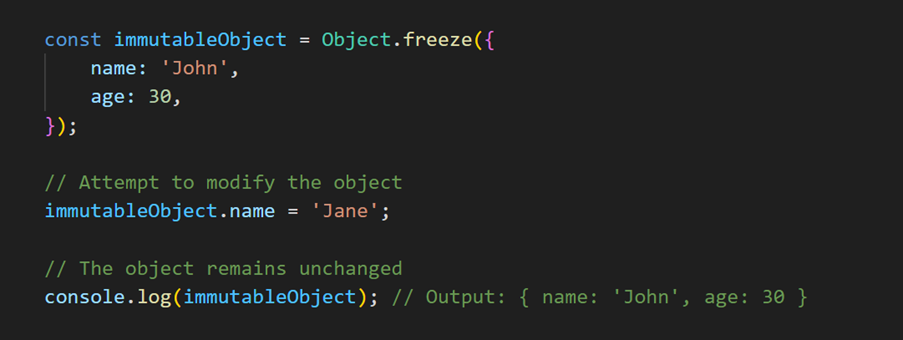
10. Explain the concept of destructuring assignment for objects and arrays.
Destructuring assignment is a syntax in JavaScript that allows you to unpack values from arrays or properties from objects into distinct variables. For arrays, you use square brackets, and for objects, you use curly braces.
Example:

61. Explain event loop in JavaScript.
The event loop is a process that continuously monitors both the call stack and the event queue and checks whether or not the call stack is empty. If the call stack is empty and there are pending events in the event queue, the event loop dequeues the event from the event queue and pushes it to the call stack. The call stack executes the event, and any additional events generated during the execution are added to the end of the event queue.
Note: The event loop allows Node.js to perform non-blocking I/O operations, even though JavaScript is single-threaded, by offloading operations to the system kernel whenever possible. Since most modern kernels are multi-threaded, they can handle multiple operations executing in the background.
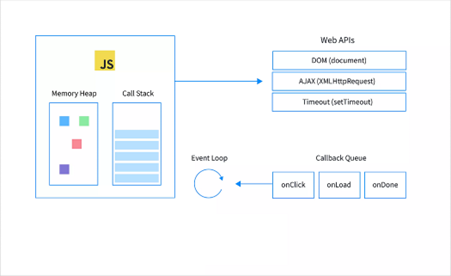
11. Explain the difference between synchronous and asynchronous functions in JavaScript.
Synchronous functions are blocking while asynchronous functions are not. In synchronous functions, statements complete before the next statement is run. As a result, programs containing only synchronous code are evaluated exactly in order of the statements. The execution of the program is paused if one of the statements take a very long time.
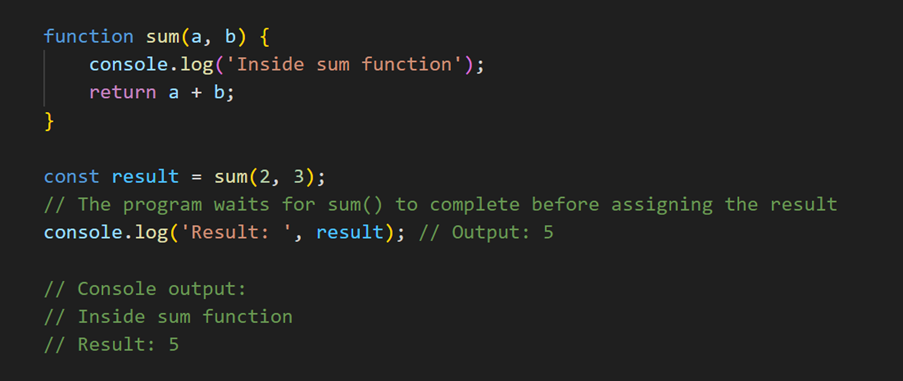
Asynchronous functions usually accept a callback as a parameter and execution continue on to the next line immediately after the asynchronous function is invoked. The callback is only invoked when the asynchronous operation is complete and the call stack is empty. Heavy duty operations such as loading data from a web server or querying a database should be done asynchronously so that the main thread can continue executing other operations instead of blocking until that long operation to complete (in the case of browsers, the UI will freeze).
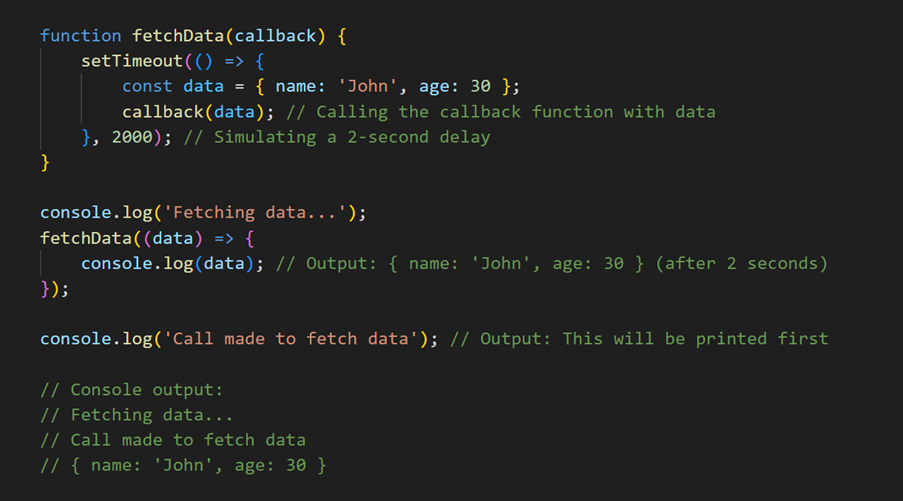
12. What are Promises and how do they work?
Promises in JavaScript are objects that represent the eventual completion (or failure) of an asynchronous operation and its resulting value. They have three states:
- pending
- fulfilled
- rejected
You can handle the results of a promise using the .then() method for success and the .catch() method for errors.
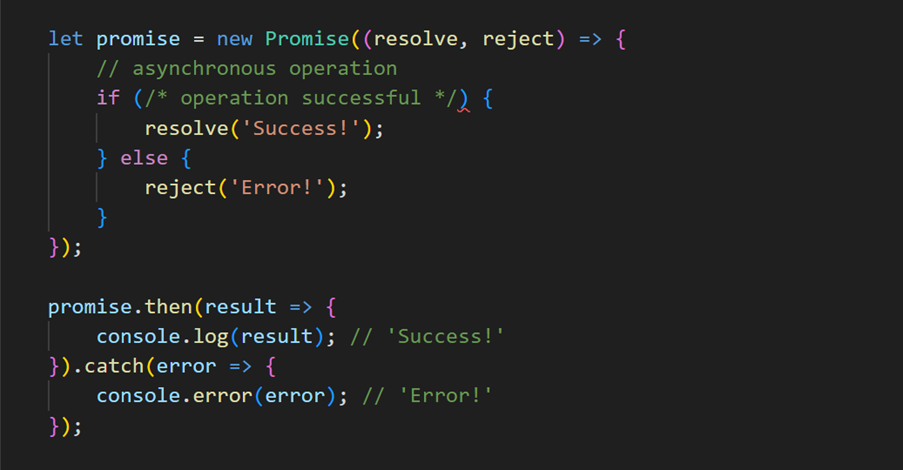
13. Explain the different states of a Promise.
A Promise in JavaScript can be in one of three states:
- pending
- fulfilled
- rejected
When a Promise is created, it starts in the pending state. If the operation completes successfully, the Promise transitions to the fulfilled state, and if it fails, it transitions to the rejected state.

14. What is async/await and how does it simplify asynchronous code?
async/await is a modern syntax in JavaScript that simplifies working with promises. By using the async keyword before a function, you can use the await keyword inside that function to pause execution until a promise is resolved. This makes asynchronous code look and behave more like synchronous code, making it easier to read and maintain.
Example:
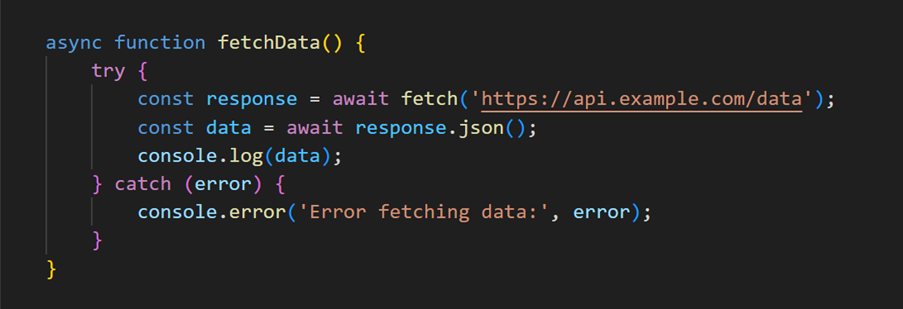
15. How do you handle errors in asynchronous operations?
To handle errors in asynchronous operations, you can use try…catch blocks with async/await syntax or .catch() method with Promises. For example, with async/await, you can wrap your code in a try…catch block to catch any errors:
Example:
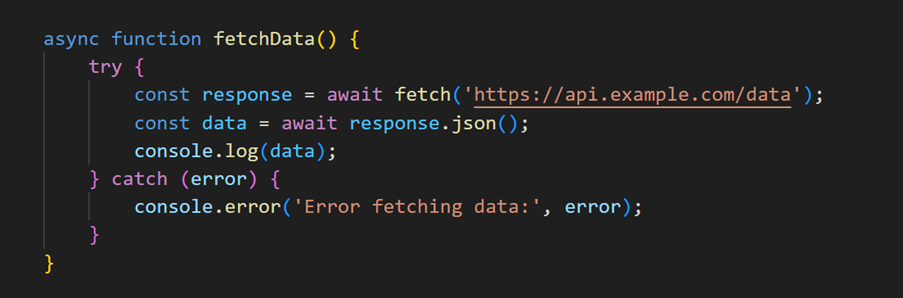
With Promises, you can use the .catch() method:

16. What is the purpose of the new keyword?
The new keyword in JavaScript is used to create an instance of a user-defined object type or one of the built-in object types that has a constructor function. When you use new, it does four things: it creates a new object, sets the prototype, binds this to the new object, and returns the new object.
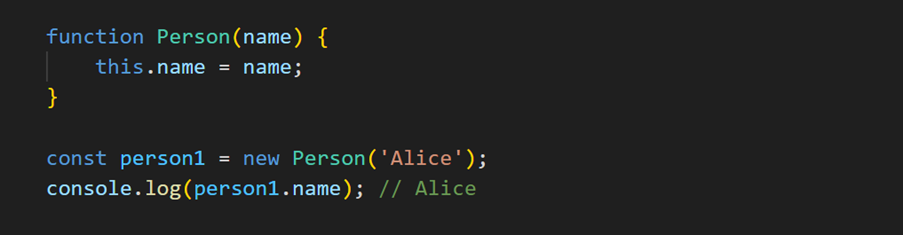
17. How do you create a constructor function?
To create a constructor function in JavaScript, define a regular function with a capitalized name to indicate it’s a constructor. Use the “this” keyword to set properties and methods. When creating an instance, use the new keyword.

18. Explain the difference between global scope, function scope, and block scope.
Global scope means variables are accessible from anywhere in the code. Function scope means variables are accessible only within the function they are declared in. Block scope means variables are accessible only within the block (e.g., within {}) they are declared in.
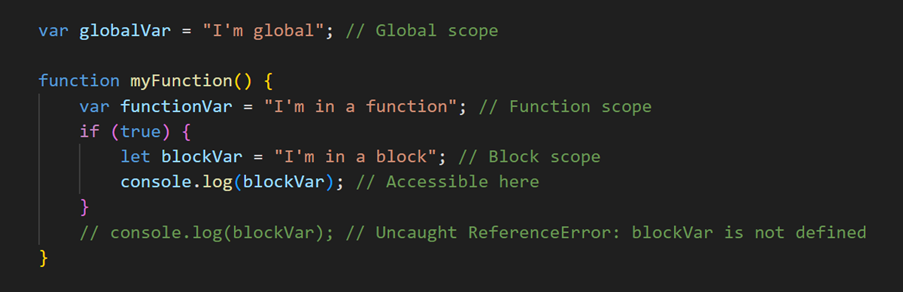
19. What is the DOM and how is it structured?
The DOM, or Document Object Model, is a programming interface for web documents. It represents the page so that programs can change the document structure, style, and content. The DOM is structured as a tree of objects, where each node represents part of the document, such as elements, attributes, and text.
20. What’s the difference between an “attribute” and a “property” in the DOM?
Attributes are defined in the HTML and provide initial values for properties. Properties are part of the DOM and represent the current state of an element. For example, the value attribute of an <input> element sets its initial value, while the value property reflects the current value as the user interacts with it.
21. Explain how “this” works in JavaScript?
In JavaScript, the keyword this refers to the object from which it was called or the context in which it is executed. Its behaviour changes based on how and where it is used. Let’s break down its usage in different contexts:
- Function Context: In a regular function, this refers to the global object (in non-strict mode) or undefined (in strict mode).
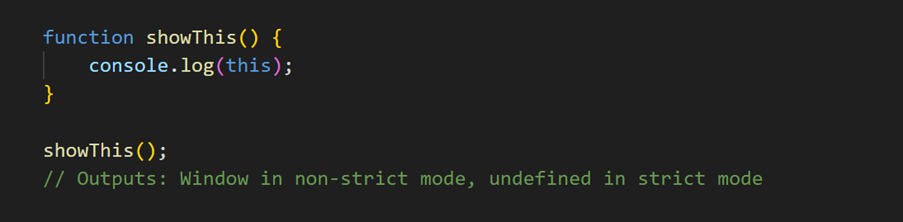
- Object Method Context: When a function is called as a method of an object, this refers to the object that the method is a property of.
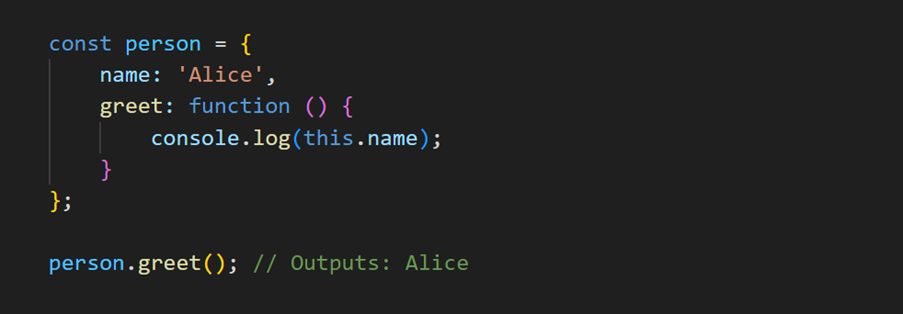
22. How do you add, remove, and modify HTML elements using JavaScript?
To add, remove, and modify HTML elements using JavaScript, you can use methods like createElement, appendChild, removeChild, and properties like innerHTML and textContent. For example, to add an element, you can create it using document.createElement and then append it to a parent element using appendChild. To remove an element, you can use removeChild on its parent. To modify an element, you can change its innerHTML or textContent
23. What are event listeners and how are they used?
Event listeners are functions those wait for specific events to occur on elements, such as clicks or key presses. They are used to execute code in response to these events. You can add an event listener to an element using the addEventListener method
Example:

24. What is the difference between innerHTML and textContent? How do you manipulate CSS styles using JavaScript?
innerHTML and textContent are both properties used to get or set the content of an HTML element, but they serve different purposes. innerHTML returns or sets the HTML markup contained within the element, which means it can parse and render HTML tags. On the other hand, textContent returns or sets the text content of the element, ignoring any HTML tags and rendering them as plain text.
Example:
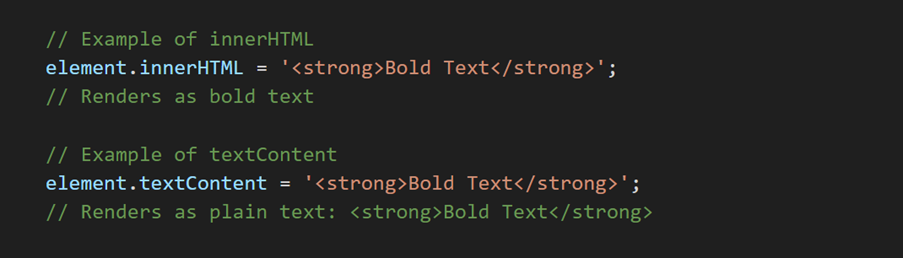
Modifying CSS
You can manipulate CSS styles using JavaScript by accessing the style property of an HTML element. For example, to change the background colour of a div element with the id myDiv, you can use:

You can also add, remove, or toggle CSS classes using the classList property:

25. What is the difference between the Window object and the Document object?
The Window object represents the browser window and provides methods to control it, such as opening new windows or accessing the browser history. The Document object represents the content of the web page loaded in the window and provides methods to manipulate the DOM, such as selecting elements or modifying their content.
26. How do you make an HTTP request using the Fetch API?
To make an HTTP request using the Fetch API, you can use the fetch function, which returns a promise. You can handle the response using .then() and .catch() for error handling.
Example:

27. What are the different ways to make an API call in JavaScript?
In JavaScript, you can make API calls using several methods. The most common ones are fetch and third-party libraries like Axios. fetch is modern and returns promises, making it easier to work with and Axios is a popular third-party library that simplifies API calls and provides additional features.
28. Explain iframe in JavaScript.
An iframe (short for inline frame) is an HTML element that allows you to embed another HTML page within the current page. In JavaScript, you can manipulate iframes to dynamically load and interact with different content.

Accessing the iframe with JavaScript
You can manipulate iframes using JavaScript to dynamically change their content, style, or behavior.
- Selecting the iframe: You can use methods like getElementById or querySelector to select an iframe element.

- Changing the Source: You can dynamically change the source (src) of an iframe

- Accessing Content within the iframe: To access or manipulate the content inside an iframe, you can use the contentWindow and contentDocument properties. Note that for security reasons, you can only access content from the same origin (same domain).

29. How do you redirect to a new page in JavaScript?
Answer: To redirect to a new page in JavaScript, you can use the window.location object. The most common methods are window.location.href and window.location.replace()
Example:
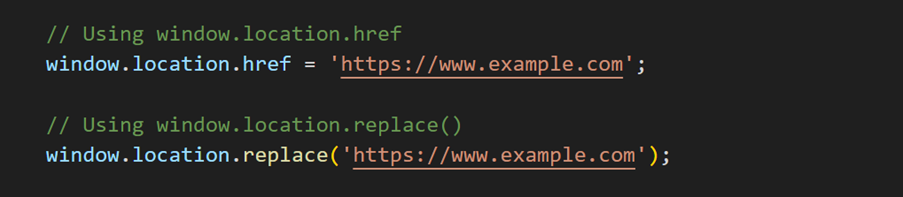
30. What is the purpose of the delete operator?
The delete operator is used to delete the property as well as its value

31. What is eval?
The eval() function evaluates JavaScript code represented as a string. The string can be a JavaScript expression, variable, statement, or sequence of statements.
Example:

32. How do you access history in JavaScript?
The window.history object contains the browser’s history. You can load previous and next URLs in the history using back() and next() methods.
Example:

33. What is the use of setInterval?
The setInterval() method is used to call a function or evaluate an expression at specified intervals (in milliseconds).
Example: Let’s log a message after 2 seconds using setInterval method

34. Why is JavaScript treated as Single threaded?
JavaScript is a single-threaded language. Because the language specification does not allow the programmer to write code so that the interpreter can run parts of it in parallel in multiple threads or processes. Whereas languages like java, go, C++ can make multi-threaded and multi-process programs.
35. What is JSON?
JSON (JavaScript Object Notation) is a lightweight format that is used for data interchanging. It is based on a subset of JavaScript language in the way objects are built in JavaScript.
36. What are the syntax rules of JSON?
Below is the list of syntax rules of JSON
- The data is in name/value pairs
- The data is separated by commas
- Curly braces hold objects
- Square brackets hold arrays
37. What is the purpose JSON stringify?
When sending data to a web server, the data has to be in a string format. You can achieve this by converting JSON object into a string using stringify() method.
Example:

38. How do you parse JSON string?
When receiving the data from a web server, the data is always in a string format. But you can convert this string value to a javascript object using parse() method.
Example:

39. Why do you need JSON?
When exchanging data between a browser and a server, the data can only be text. Since JSON is text only, it can easily be sent to and from a server, and used as a data format by any programming language.
40. What is the purpose of clearTimeout method?
The clearTimeout() function is used in javascript to clear the timeout which has been set by setTimeout()function before that. i.e, The return value of setTimeout() function is stored in a variable and it’s passed into the clearTimeout() function to clear the timer.
Example: The below setTimeout method is used to display the message after 3 seconds. This timeout can be cleared by the clearTimeout() method.
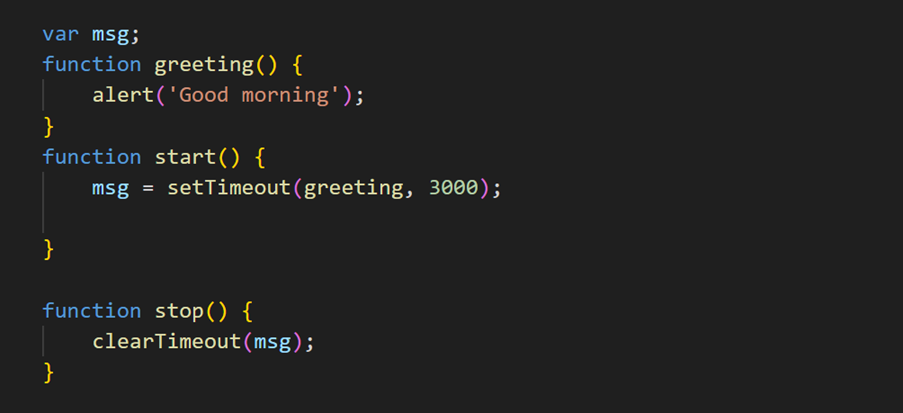
41. How do you validate an email in JavaScript?
You can validate an email in JavaScript using regular expressions.
Example: The regex pattern /^[^\s@]+@[^\s@]+\.[^\s@]+$/ is used to match a basic email structure.
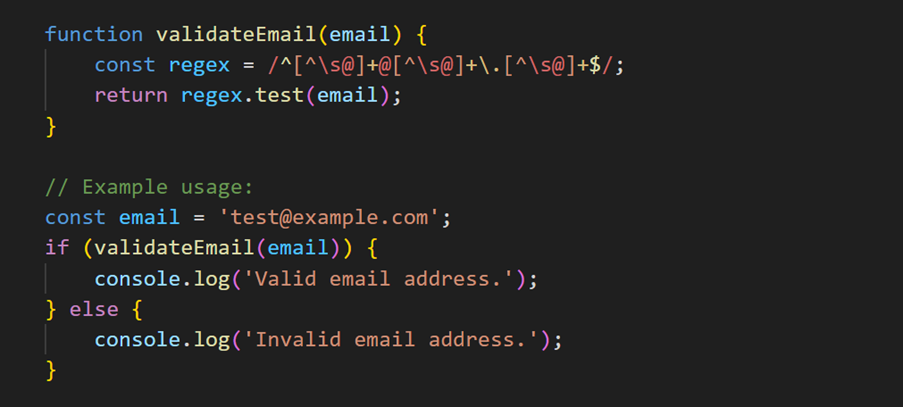
42. How do you test for an empty object?
The Object.keys() method returns an array of a given object’s own enumerable property names. If the length of this array is 0, the object is empty.
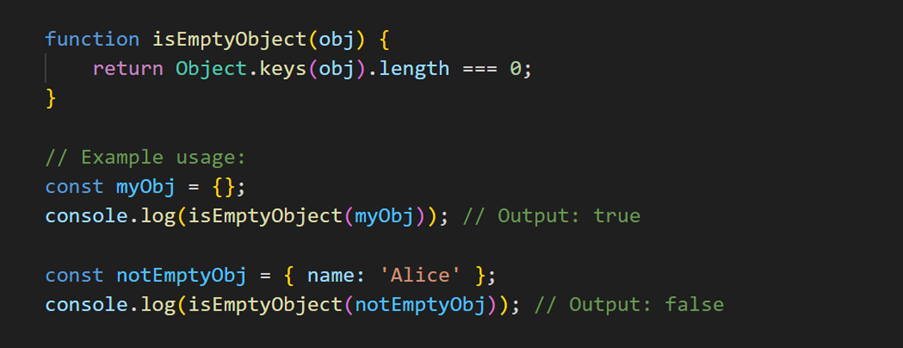
43. How do you make first letter of the string in an uppercase?
You can create a function which uses a chain of string methods such as charAt, toUpperCase and slice methods to generate a string with the first letter in uppercase
Example:

44. How do you display the current date in JavaScript?
You can use new Date() to generate a new Date object containing the current date and time.
Example: Let’s display the current date in mm/dd/yyyy
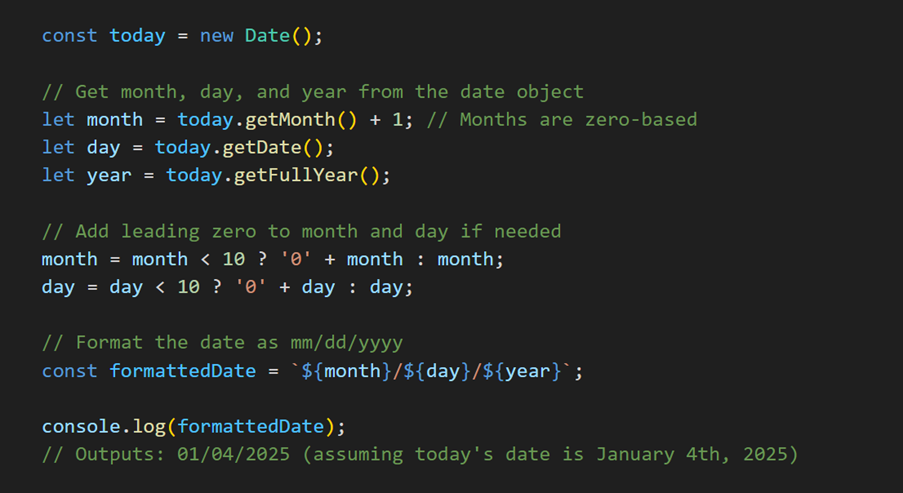
45. How do you compare two date objects?
You need to use date.getTime() method to compare date values instead of comparison operators (==, !=, ===, and !== operators)
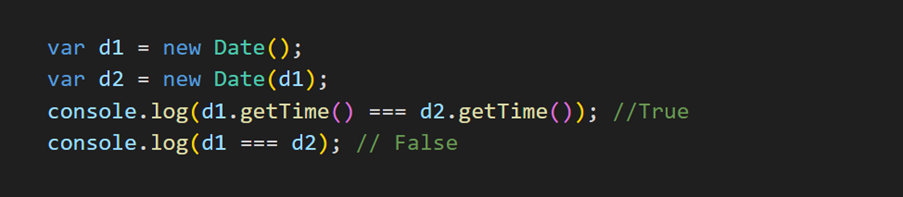
46. How do you trim a string in JavaScript?
JavaScript provided a trim method on string types to trim any whitespaces present at the beginning or ending of the string.

47. How do you add a key value pair in JavaScript Object?
Let’s take a simple object to explain these solutions.

- Using dot notation: This solution is useful when you know the name of the property

- Using square bracket notation: This solution is useful when the name of the property is dynamically determined

48. What are the benefits of keeping declarations at the top?
It is recommended to keep all declarations at the top of each script or function. The benefits of doing this are
- Gives cleaner code
- It provides a single place to look for local variables
- Easy to avoid unwanted global variables
- It reduces the possibility of unwanted re-declarations
49. What are the benefits of initializing variables?
It is recommended to initialize variables because of the below benefits:
- It gives cleaner code
- It provides a single place to initialize variables
- Avoid undefined values in the code
50. What is the purpose of the console object in JavaScript?
The console object in JavaScript is primarily used for debugging and logging information during development. It provides a set of methods that allow you to output messages to the web browser’s console.